As an Intune administrator, you likely use PowerShell for automation and management tasks. With Microsoft’s deprecation of the AzureAD PowerShell module, you might be wondering about the new Microsoft Entra PowerShell module and whether it’s something you need in your automation toolkit arsenal.
In this post, we’ll analyse the Entra PowerShell module’s authentication implementation and discuss whether it’s necessary for your Intune automation needs if you’re already using the Microsoft.Graph.Authentication module, part of the Microsoft Graph SDK, to handle your tokens.
tl;dr For Intune administrators doing automation, using the Microsoft.Graph.Authentication module directly might be a better approach still. But read on for funsies….
Why should Intune Admins Care?
I am going to take a small gamble and suggest the deprecation of AzureAD PowerShell module may not have a huge impact on your existing Intune automation scripts. It’s highly likely that you have already transitioned to using the Microsoft Graph SDK after the deprecation of another previously popular PowerShell module, MSAL.PS.
Here are the 3 amigo’s in my lab.

While the new Entra PowerShell module serves as a replacement for general Azure AD management, as an Intune administrator, you’re probably more interested in its authentication capabilities for your Intune automation scripts. Let’s examine whether this new module adds value to your specific use case.
AzureAD Migration Considerations
If you are refactoring your scripts and moving away from the AzureAD PowerShell, you have two options as I see it (for authentication at least):-
- Microsoft Graph PowerShell SDK
- Entra PowerShell Module
The key difference as far as Intune admins are concerned? Not a lot of cheese in it so far, to be fair. Lets dive deeper into authentication – thats why most of us Intune nerds are here is my guess…
Entra Authentication Cmdlets
1. Connect-Entra Implementation
The core authentication function in the Microsoft Entra PowerShell module is Connect-Entra
. Looking at the source code, we can see that this is actually a wrapper around Connect-MgGraph
from the Microsoft.Graph.Authentication module:-
function Connect-Entra { [CmdletBinding(DefaultParameterSetName = 'UserParameterSet')] param ( # ... parameter definitions ... ) begin { $wrappedCmd = $ExecutionContext.InvokeCommand.GetCommand( 'Microsoft.Graph.Authentication\Connect-MgGraph', [System.Management.Automation.CommandTypes]::Cmdlet ) $scriptCmd = { & $wrappedCmd @PSBoundParameters } $steppablePipeline = $scriptCmd.GetSteppablePipeline($myInvocation.CommandOrigin) $steppablePipeline.Begin($PSCmdlet) } }
Ah Ha! I hear you cry. We have fake witchery in our presence. Its like the other kid in the classroom stealing your test answers and claiming they did all the work. Grabbing a token during the authentication flow when using the Connect-Entra
cmdlet is actually using the Connect-MgGraph
cmdlet in the Microsoft.Entra.Authentication PowerShell module to do the heavy lifting.
The cmdlet relies on Microsoft.Graph.Authentication for its core functionality, passing through all parameters directly to Connect-MgGraph
.
Ok, some of you want proof…
Get-Command Connect-Entra | Format-List *
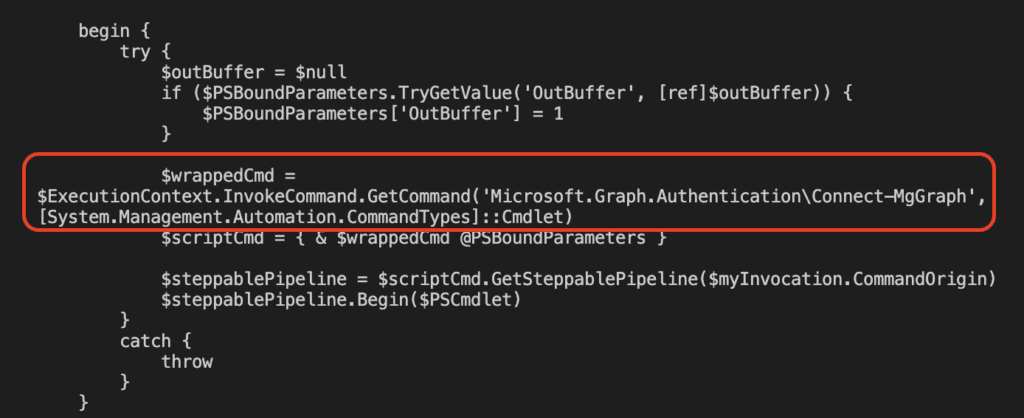
Trace-Command -Name ParameterBinding -Expression { Connect-Entra -Verbose } -PSHost Connect-Entra -Verbose (Get-Command Connect-Entra).ScriptBlock
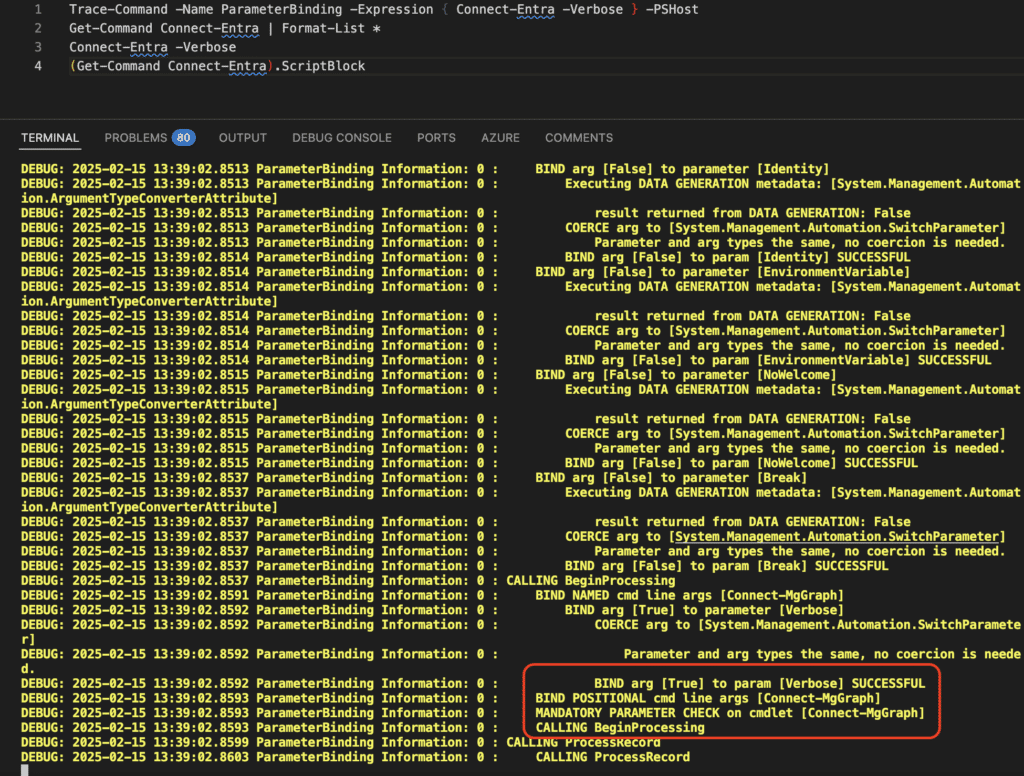
2. Disconnect-Entra Implementation
Similarly, Disconnect-Entra
is a direct wrapper around Disconnect-MgGraph
powershellCopyfunction Disconnect-Entra {
[CmdletBinding(DefaultParameterSetName = 'GetQuery')]
param ()
Disconnect-MgGraph
}
3. Custom Headers and Graph Requests
The Entra module also uses Invoke-MgGraphRequest
for API calls. Here is an example from the module for the cmdlet Revoke-EntraSignedInUserAllRefreshToken
function Revoke-EntraSignedInUserAllRefreshToken { [CmdletBinding(DefaultParameterSetName = '')] param () PROCESS { $params = @{} $customHeaders = New-EntraCustomHeaders -Command $MyInvocation.MyCommand $response = (Invoke-GraphRequest -Headers $customHeaders -Uri 'https://graph.microsoft.com/v1.0/me/revokeSignInSessions' -Method POST).value if($response){ $responseType = New-Object Microsoft.Graph.PowerShell.Models.ComponentsMwc6EoResponsesRevokesigninsessionsresponseContentApplicationJsonSchema $responseType.Value= $response $responseType } } }
$response = (Invoke-GraphRequest -Headers $customHeaders -Uri 'https://graph.microsoft.com/v1.0/me/revokeSignInSessions' -Method POST).value
Implications for Intune Automation
If you’re primarily using a PowerShell module for authentication in your Intune automation scripts, there are some important considerations:
If your scripts mainly use Invoke-MgGraphRequest
, you can continue using Microsoft.Graph.Authentication directly. Here is an example of how you might be doing that using only the Microsoft.Graph.Authentication module.
# Using Microsoft.Graph.Authentication directly Connect-MgGraph -Scopes "DeviceManagementConfiguration.ReadWrite.All" $token = Get-MgContext | Select-Object -ExpandProperty AccessToken # Make direct Graph API calls $headers = @{ "Authorization" = "Bearer $token" "Content-Type" = "application/json" } Invoke-RestMethod -Uri "https://graph.microsoft.com/beta/deviceManagement/deviceConfigurations" -Headers $headers
Am I in love yet?
I want to fall in love with the Entra PowerShell module, and I do believe there is a place for it, but I’m not keen on using one SDK that relies on another SDK – there are too many variables at play to hope that the development on one SDK is maintained at the same pace as development on the other.
The 2 main advantages of using the Entra module is:
- Backwards compatibility with AzureAD module commands through aliases
- Simplified parameter sets for common Entra ID operations
Authentication Methods using Entra.Authentication
The Entra.Authentication module supports various authentication scenarios. Here are the key methods with real-world examples:
Available Commands in the Module
The Entra PowerShell module provides these core cmdlets for authentication management:
# 1. Interactive Browser Authentication (Most common for interactive scripts) Connect-Entra # or with specific scopes Connect-Entra -Scopes "DeviceManagementConfiguration.ReadWrite.All" # 2. Device Code Flow (Good for remote/headless scenarios) Connect-Entra -UseDeviceCode # 3. Service Principal with Certificate Connect-Entra -ClientId "YOUR_APP_ID" ` -TenantId "YOUR_TENANT_ID" ` -CertificateThumbprint "CERT_THUMBPRINT" # 4. Service Principal with Client Secret $clientSecretCredential = Get-Credential Connect-Entra -ClientId "YOUR_APP_ID" ` -TenantId "YOUR_TENANT_ID" ` -ClientSecretCredential $clientSecretCredential # 5. Managed Identity (for Azure resources) Connect-Entra -Identity # 6. Access Token (if you already have one) $token = ConvertTo-SecureString "YOUR_TOKEN" -AsPlainText -Force Connect-Entra -AccessToken $token
The core authentication cmdlets you might use if there is a case to use the Entra modules in your automation scripts are:-
# Core Authentication Commands Connect-Entra # Connect to Microsoft Entra ID Disconnect-Entra # End the session Get-EntraContext # Get current authentication context
Working with Context
The module Get-EntraContext
allows you to check your authentication context. Again, we are simply calling Get-MgContext under the proverbial hood.
Connect-Entra $entraContext = Get-EntraContext $graphContext = Get-MgContext # Compare the outputs - they'll be identical except for the added Entra properties $entraContext | Format-List $graphContext | Format-List
Do they look similar? š
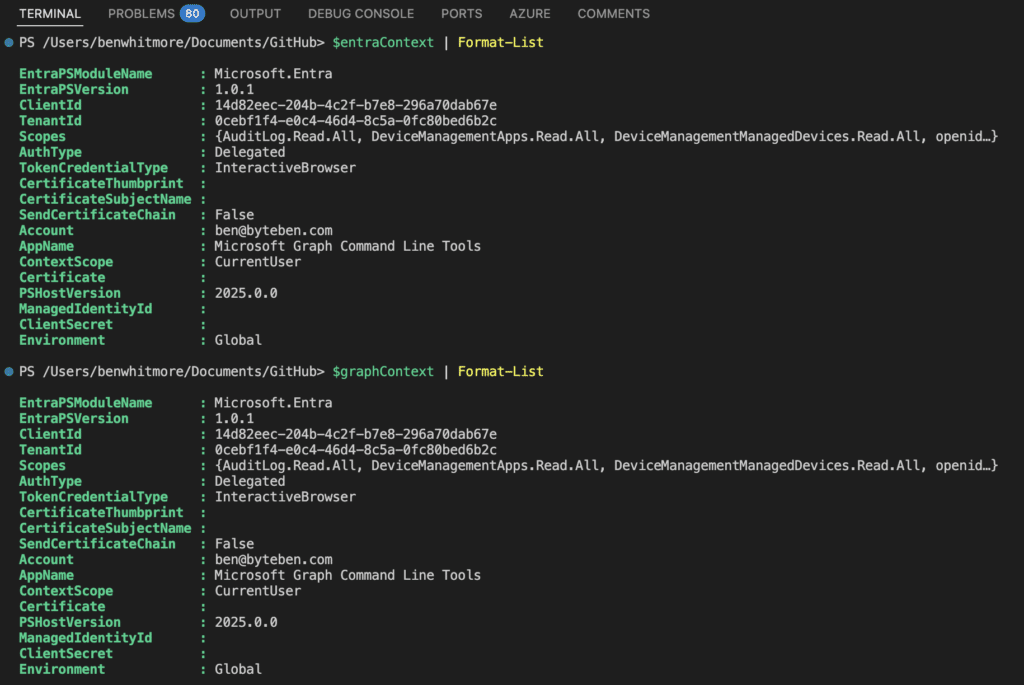
Migration from AzureAD Module
The Entra module provides aliases for AzureAD cmdlets. If you are interested in the new aliases, we can look at the Enable-EntraAzureADAlias
function, as it defines aliases for any AzureAD cmdlets you may have used previously. Although I don’t cover them that much in the blog, here’s what that looks like in the Entra module PSM1:-
# From the PSM1 file function Enable-EntraAzureADAlias { Set-Alias -Name Revoke-AzureADUserAllRefreshToken -Value Revoke-EntraUserAllRefreshToken -Scope Global -Force Set-Alias -Name Revoke-AzureADSignedInUserAllRefreshToken -Value Revoke-EntraSignedInUserAllRefreshToken -Scope Global -Force Set-Alias -Name Get-AzureADMSAdministrativeUnit -Value Get-EntraUnsupportedCommand -Scope Global -Force Set-Alias -Name Connect-AzureAD -Value Connect-Entra -Scope Global -Force Set-Alias -Name Disconnect-AzureAD -Value Disconnect-Entra -Scope Global -Force # ... many more aliases ... }
To use these aliases in your existing scripts:
# Old script Import-Module AzureAD Connect-AzureAD Get-AzureADUser # Modified script - just change the import Import-Module Microsoft.Entra # The rest of the script remains the same Connect-AzureAD # This now calls Connect-Entra Get-AzureADUser # This now calls the Graph equivalent
Breaking Changes and Considerations
When migrating from AzureAD PowerShell, be aware of these key changes:
- Parameter Changes
- Graph SDK uses consistent parameter names across cmdlets
- Some parameters from AzureAD module have been renamed. e.g.
-ObjectId
becomes-UserId
in some cases
- Return Object Differences
- Graph SDK returns different object types
- Properties might have different names or structures
- More consistent property naming across different object types
- Authentication Flow Changes
- Modern authentication is now the default
- Better support for automation scenarios
- Improved token handling and renewal
Why should Intune Admins not use the Entra module?
While looking at the Entra PowerShell module implementation, I have come to the conclusion that there might be several reasons why you might want to skip using it in favour of using the Microsoft.Graph.Authentication module directly:
- Additional Layer of Abstraction
- The Entra module is essentially a wrapper around Microsoft.Graph.Authentication
- It adds another dependency to your scripts without significant benefits
- Most functions are simple pass-throughs to Graph cmdlets
- Alias Limitations
- While the aliases provide backwards compatibility, they’re not a long-term solution
- Maintaining scripts with aliases makes them less readable and maintainable
- Performance and Intune-Specific Requirements
- Using direct Graph authentication removes an unnecessary layer
- Fewer module dependencies mean faster script loading
- Direct Graph calls give you more control over the request pipeline
- Intune management often requires beta endpoint access, which is more straightforward with direct Graph calls
Conclusion
For Intune administrators focused on device management and automation, my thoughts and guidance is clear – migrate to Graph SDK or continue to use it. While the Entra PowerShell module provides a transitional path through aliases and compatibility features, there are compelling reasons to skip it and use Graph SDK directly:
My key takeaway is this… While the Entra module provides a comfortable bridge for those familiar with AzureAD cmdlets, investing time in learning Graph SDK directly will provide better long-term value for your Intune automation needs.
Add comment